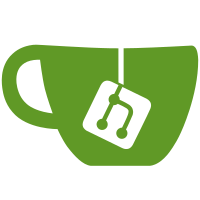
Signed-off-by: Alexander Onnikov <Alexander.Onnikov@xored.com>
7.7 KiB
Huly Platform API Client
A TypeScript client library for interacting with the Huly Platform API.
Installation
In order to be able to install required packages, you will need to obtain GitHub access token. You can create a token by following the instructions here.
npm install @hcengineering/api-client
Authentication
There are two ways to connect to the platform, using email and password, or using token.
Parameters:
url
: URL of the Huly instanceoptions
: Connection optionsworkspace
: Name of the workspace to connect totoken
: Optional authentication tokenemail
: Optional user emailpassword
: Optional user passwordconnectionTimeout
: Optional connection timeoutsocketFactory
: Optional socket factory
Using Email and Password
const client = await connect('http://localhost:8087', {
email: 'user1',
password: '1234',
workspace: 'ws1'
})
...
await client.close()
Using Token
const client = await connect('http://localhost:8087', {
token: '...',
workspace: 'ws1'
})
...
await client.close()
Example usage
Fetch API
The client provides two main methods for retrieving documents: findOne
and findAll
.
findOne
Retrieves a single document matching the query criteria.
Parameters:
_class
: Class of the object to find, results will include all subclasses of the targe classquery
: Query criteriaoptions
: Find optionslimit
: Limit the number of results returnedsort
: Sorting criterialookup
: Lookup criteriaprojection
: Projection criteriatotal
: If specified total will be returned
Example:
import contact from '@hcengineering/contact'
...
const person = await client.findOne(
contact.class.Person,
{
_id: 'person-id'
}
)
findAll
Retrieves multiple document matching the query criteria.
Parameters:
_class
: Class of the object to find, results will include all subclasses of the targe classquery
: Query criteriaoptions
: Find optionslimit
: Limit the number of results returnedsort
: Sorting criterialookup
: Lookup criteriaprojection
: Projection criteriatotal
: If specified total will be returned
Example:
import { SortingOrder } from '@hcengineering/core'
import contact from '@hcengineering/contact'
..
const persons = await client.findAll(
contact.class.Person,
{
city: 'New York'
},
{
limit: 10,
sort: {
name: SortingOrder.Ascending
}
}
)
Documents API
The client provides three main methods for managing documents: createDoc
, updateDoc
, and removeDoc
. These methods allow you to perform CRUD operations on documents.
createDoc
Creates a new document in the specified space.
Parameters:
_class
: Class of the objectspace
: Space of the objectattributes
: Attributes of the objectid
: Optional id of the object, if not provided, a new id will be generated
Example:
import contact, { AvatarType } from '@hcengineering/contact'
..
const personId = await client.createDoc(
contact.class.Person,
contact.space.Contacts,
{
name: 'Doe,John',
city: 'New York',
avatarType: AvatarType.COLOR
}
)
updateDoc
Updates exising document.
Parameters:
_class
: Class of the objectspace
: Space of the objectobjectId
: Id of the objectoperations
: Attributes of the object to update
Example:
import contact from '@hcengineering/contact'
..
await client.updateDoc(
contact.class.Person,
contact.space.Contacts,
personId,
{
city: 'New York',
}
)
removeDoc
Removes exising document.
Parameters:
_class
: Class of the objectspace
: Space of the objectobjectId
: Id of the object
Example:
import contact from '@hcengineering/contact'
..
await client.removeDoc(
contact.class.Person,
contact.space.Contacts,
personId
)
Collections API
addCollection
Creates a new attached document in the specified collection.
Parameters:
_class
: Class of the object to createspace
: Space of the object to createattachedTo
: Id of the object to attach toattachedToClass
: Class of the object to attach tocollection
: Name of the collection containing attached documentsattributes
: Attributes of the objectid
: Optional id of the object, if not provided, a new id will be generated
Example:
import contact, { AvatarType } from '@hcengineering/contact'
..
const personId = await client.createDoc(
contact.class.Person,
contact.space.Contacts,
{
name: 'Doe,John',
city: 'New York',
avatarType: AvatarType.COLOR
}
)
await client.addCollection(
contact.class.Channel,
contact.space.Contacts,
personId,
contact.class.Person,
'channels',
{
provider: contact.channelProvider.Email,
value: 'john.doe@example.com'
}
)
updateCollection
Updates exising attached document in collection.
Parameters:
_class
: Class of the object to updatespace
: Space of the object to updateobjectId
: Space of the object to updateattachedTo
: Id of the parent objectattachedToClass
: Class of the parent objectcollection
: Name of the collection containing attached documentsattributes
: Attributes of the object to update
Example:
import contact from '@hcengineering/contact'
..
await client.updateCollection(
contact.class.Channel,
contact.space.Contacts,
channelId,
personId,
contact.class.Person,
'channels',
{
city: 'New York',
}
)
removeCollection
Removes exising attached document from collection.
Parameters:
_class
: Class of the object to removespace
: Space of the object to removeobjectId
: Space of the object to removeattachedTo
: Id of the parent objectattachedToClass
: Class of the parent objectcollection
: Name of the collection containing attached documents
Example:
import contact from '@hcengineering/contact'
..
await client.removeCollection(
contact.class.Channel,
contact.space.Contacts,
channelId,
personId,
contact.class.Person,
'channels'
)
Mixins API
The client provides two methods for managing mixins: createMixin
and updateMixin
.
createMixin
Creates a new mixin for a specified document.
Parameters:
objectId
: Id of the object the mixin is attached toobjectClass
: Class of the object the mixin is attached toobjectSpace
: Space of the object the mixin is attached tomixin
: Id of the mixin type to updateattributes
: Attributes of the mixin
import contact, { AvatarType } from '@hcengineering/contact'
..
const personId = await client.createDoc(
contact.class.Person,
contact.space.Contacts,
{
name: 'Doe,John',
city: 'New York',
avatarType: AvatarType.COLOR
}
)
await client.createMixin(
personId,
contact.class.Person,
contact.space.Contacts,
contact.mixin.Employee,
{
active: true,
position: 'CEO'
}
)
updateMixin
Updates an existing mixin.
Parameters:
objectId
: Id of the object the mixin is attached toobjectClass
: Class of the object the mixin is attached toobjectSpace
: Space of the object the mixin is attached tomixin
: Id of the mixin type to updateattributes
: Attributes of the mixin to update
import contact, { AvatarType } from '@hcengineering/contact'
..
const person = await client.findOne(
contact.class.Person,
{
_id: 'person-id'
}
)
await client.updateMixin(
personId,
contact.class.Person,
contact.space.Contacts,
contact.mixin.Employee,
{
active: false
}
)