mirror of
https://github.com/hcengineering/platform.git
synced 2025-02-02 08:51:11 +00:00
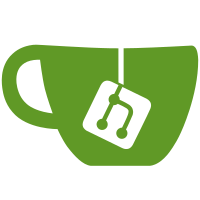
Signed-off-by: Denis Bykhov <80476319+BykhovDenis@users.noreply.github.com> Co-authored-by: Alexander Platov <sas_lord@mail.ru>
36 lines
902 B
TypeScript
36 lines
902 B
TypeScript
export function getTime (time: number): string {
|
|
let options: Intl.DateTimeFormatOptions = { hour: 'numeric', minute: 'numeric' }
|
|
if (!isCurrentYear(time)) {
|
|
options = {
|
|
year: '2-digit',
|
|
month: 'numeric',
|
|
day: 'numeric',
|
|
...options
|
|
}
|
|
} else if (!isToday(time)) {
|
|
options = {
|
|
month: 'numeric',
|
|
day: 'numeric',
|
|
...options
|
|
}
|
|
}
|
|
|
|
return new Date(time).toLocaleString('default', options)
|
|
}
|
|
|
|
export function isToday (time: number): boolean {
|
|
const current = new Date()
|
|
const target = new Date(time)
|
|
return (
|
|
current.getDate() === target.getDate() &&
|
|
current.getMonth() === target.getMonth() &&
|
|
current.getFullYear() === target.getFullYear()
|
|
)
|
|
}
|
|
|
|
export function isCurrentYear (time: number): boolean {
|
|
const current = new Date()
|
|
const target = new Date(time)
|
|
return current.getFullYear() === target.getFullYear()
|
|
}
|